Webpack Vue.js CLI
Use vue-cli to download vuejs, webpack and template dependencies and create the project in the root of our plugin folder. Navigate to the wp-content/plugins directory.
Update or install globally as needed.
18 Nov 2018 Using Vue.js with WordPress allows developers to create modern web apps on top of the most popular CMS in the world. In fact, with the addition of the REST API, WordPress has become a complete application platform for building web apps with a PHP/WP back-end and a JavaScript front-end. The Vue application will then be bootstrapped into the #app div. That’s about it for the WordPress part of the theme. The Vue part of the theme can be found in the rest-theme folder. The Vue application is created in the src/main.js file. Here we create the main App component with a simple template. Vue can scale up to be used for full-blown single page applications, but you can also use it to add small bits of interactivity to sites, pages, or plugins where in the past you may have used jQuery.
Change to the wordpress plugins directory and use vue-cli to install the webpack-simple template into the new wp-api-vuejs-poc folder. For example,
Here is a tree view of the plugin folders and files at this point. Skip ahead and download or browse the source code if you want.
- wp-api-vuejs-poc
- src
- assets
- App.vue
- main.js
- .bablerc
- .gitignore
- class-api-vpoc-page.php
- index.html
- package.json
- README.md
- webpack.config.js
- wp-api-vuejs-poc.php
- src
Change back to our new plugin directory, for example wp-api-vuejs-poc.
Install node modules with npm install
or npm i
.
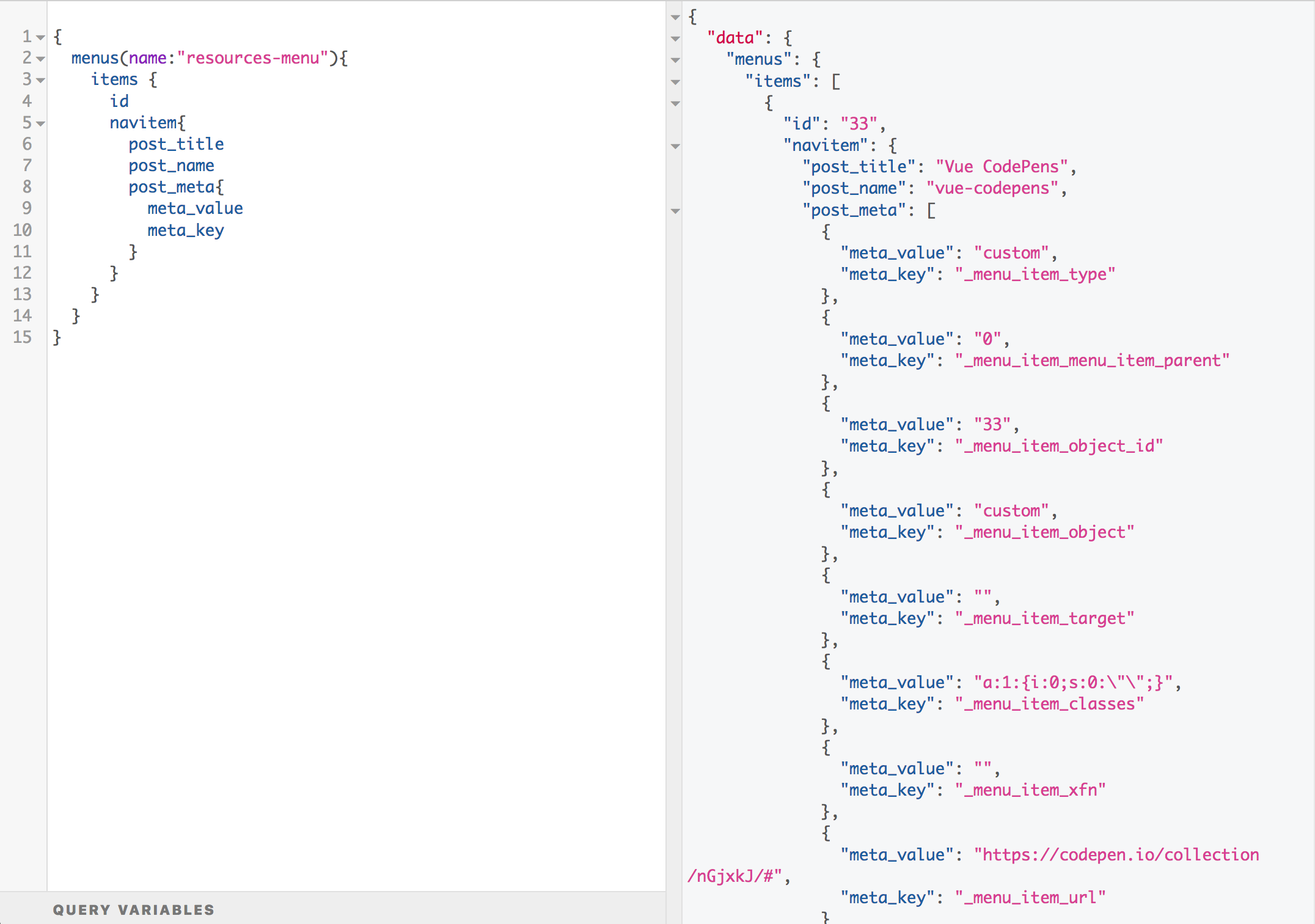
Development Build
Since the Vuejs app is running in WordPress, we do not need the webpack-dev-server
that is included in the vue-cli webpack-simple template.
Uninstall the webpack-dev-server
package with npm uninstall
using --save-dev
option to update the package.json npm manifest.
Update the package.json
scripts property so the npm run dev
command runs webpack development compilation with watch mode enabled. Watch mode recompiles after every change is saved.
REPLACE
WITH
Vue Resource
We are using vue-resource to make the wp-api requests and handle responses. Install with --save-dev
to update the package.json npm manifest.
Page Slug
A front end page template and respective slug is needed to output the app in WordPress on the front end. Last week, I wrote a quick post with details on how to do this. The slug value, api-test
is set in the class-api-vpoc-page.php file.
This proof-of-concept was tested with WordPress permalink settings set to Post name.
Let’s do a build to make sure everything is hooked up properly at this point.
After the build, you should now have a dist
folder in the plugin root containing the javascript and any other static assets.
Login to WordPress and Activate the plugin. Then navigate to the front end page, for example, http://wordpress.dev:8080/api-test/. You should see the default vue-cli template content.
Default App build
Vue App Component
Open up the src/App.vue
file and replace the contents with the following containing our post form, vue model and submit handler.
App.vue
Open up the src/main.js
file and replace the contents with the following javascript. Included is the Vue resource settings for the X-WP-Nonce
WordPress security token in the request header.
main.js
Build the App
Manually refresh the api-test page to verify the build. You should now have a working front end form to Submit a Post.
Submit Post Form
Versions that were used in this tutorial.
- WordPress 4.7
- Vue v2.1.0
- Vue-cli v2.6.0
- Vue-resource v1.0.3
- Webpack v2.1.0-beta.25
Source Code
The next page covers using axios, jQuery and other methods for making web requests instead of vue-resource.
Axios
Axios is a popular HTTP client library that covers almost everything vue-resource provides with a similar API.
Install Axios
To simplify using Axios in our Vue app, install the vue-axios wrapper for Vue integration.
Code Updates
Update src/main.js
as follows.
main.js
Update src/App.vue
as follows.
REPLACE
WITH
Rebuild the App
Uninstall vue-resource
Refresh the api-test page and Submit a post to confirm the build and app are working as expected.
The axios-p01 tag in the wp-api-vuejs-poc
source code repository on GitHub has been updated to use axios instead of vue-resource for ajax requests:
Source Code
jQuery
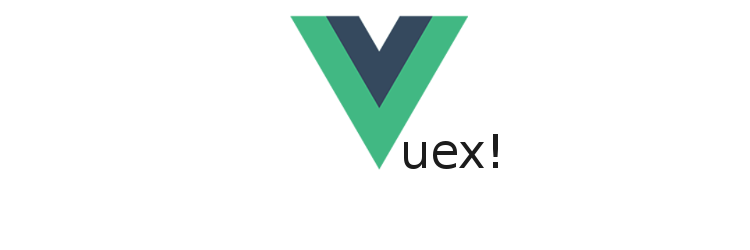
Since jQuery is loaded by default in WordPress, we can use its ajax asynchronous HTTP method for the API request.
Code Updates
Remove all of the vue-resource code in src/main.js
.
main.js
Replace the script block code in src/App.vue
as follows.
The global jQuery object needs to be available to the app to use its ajax method. Add the externals
option to the webpack.config.js
and specify jquery so it can be declared as a dependency.
The jquery-p01 tag in the wp-api-vuejs-poc
source code repository on GitHub has been updated with these changes. The vue-resource plugin is removed and replaced with the ajax method available in the external jQuery library loaded by default in WordPress:
Source Code
Part II
Part 2 of this series covers adding the following to the Vue.js front end WordPress application.
- List posts submitted by the current user
- Select from the list to edit a post
- Delete a post from the list
Resources
WordPress, Vue and Nuxt — an SEO love story
I wrote a surprisingly popular article a few months back titled: “Vue.js & the WordPress REST API on a large content site” — I received a number of comments on that article asking for further information on how we got the SEO working.
So here goes 🙂
This tutorial demonstrates, very simply, how one would make use of the WordPress SEO Plugin by Yoast to provide SEO Title and Metadata to a Vue.js front end over the WordPress REST API.
There are a few pieces to this puzzle so I’ll try to be as clear as possible, without wasting anyone’s time and go through each piece in sufficient, but not laborious, detail.
I’ve created the simplest possible example to demonstrate what’s involved but you’ll need quite a lot more to get it all working smoothly in a production environment which I’ll touch on briefly at the end.
We’ll go through the following steps:
- Setup a Vue.js (Nuxt) frontend which can display single posts.
- Setup a WordPress API that serves post data over a restful endpoint.
- Add Yoast SEO Plugin data to that endpoint.
- Connect the Vue JS frontend to the API to pull the post and SEO meta data.
This tutorial assumes the following:
- An intermediate knowledge of WordPress (PHP) development.
- An understanding of Javascript development and some of what goes with that: NPM etc
- Light familiarity with Yoast’s SEO Plugin for WordPress.
- An interest in using Vue.js.
Setup a Vue.js (Nuxt) frontend which can display single posts.
Choose a directory on your machine and create a new nuxt project like so:
Follow the steps and configure it however you’d like — I went with NPM over Yarn, no linting and no tests for the purposes of this lil demo.
Run the following to see your site on: http://localhost:3000
And voila — You’ll see your vue-tiful new project running!
After that you’ll want to navigate to the “pages” directory and create a new folder called “post”. Inside that folder create two files: index.vue and _id.vue.
The index file will be used to disaply the listing page for posts which we won’t be covering in this tutorial and the _id.vue file will be used to display the individual posts.
In the ‘_id.vue’ file add the following code for now:
Then visit this here: http://localhost:3000/post/1
There we go, a lovely little posts template showing the same static data over and over again no matter what ID is entered into the URL.
Next we will setup a WordPress API that serves post data.
I install WP locally via Docker but it’s totally your call how you wanna do the installation bit. Once you’ve got a site setup we work with the WordPress Rest API to serve the post data — with some additional SEO metadata using Yoast’s SEO Plugin.
WordPress, by default, provides an API endpoint for posts.
You can visit this here:http://your-local-wp-site-url/wp-json/wp/v2/posts/POST_ID
My exact one was: http://devapi.seotest.com:20080/wp-json/wp/v2/posts/1
This will produce a response which looks something like the following:
There you go! You have an API endpoint setup which returns post data and you didn’t even have to write any code!
Add Yoast SEO data to those posts.
The next part is also pretty straight forward. We need to add Yoast’s SEO Meta data to this response.
First things first — visit your plugins page and install the Yoast SEO plugin.
I’m only going to focus on the Title and Meta Description to keep this post from getting too long but the rest of the fields follow a very similar pattern.
Add the following code to your functions.php:
What this does is the following:
Hooks into the ‘rest_api_init’ function to register two custom fields. Using ‘rest_api_init’ instead of ‘init’ so that this code only runs on API requests and not regular post requests.
Wordpress Vuejs Tutorial
Specifies the callback function for fetching the two fields as ‘get_seo_meta_field’ which simply takes the object ID (the post in this case) and the field name (either ‘_yoast_wpseo_title’ or ‘_yoast_wpseo_metadesc’) and returns the value of that post meta field.
Wonderful! Now we have a posts API endpoint that returns Yoast SEO Meta data! Woop Woop!
You can find the full description for how to add additional meta fields to endpoints here: https://v2.wp-api.org/extending/modifying
Connect the Vue JS app to pull post data
This is the final step — we need to tell the Vue Frontend to fetch the Post Data for each post from the WordPress API. We do this as follows:
We’re going to use Axios for this so in your _id.vue file add the following in the <script> tags.
What’s going on here?
- We’re importing Axios into the file.
- We’re using Nuxt’s ‘asyncData’ method to perform our Axios request.
- We’re using a dynamic ID parameter so that we can pull different posts by visiting different URLs.
- We’re adding the returned post data into a ‘post’ object that we’ve created.
We can now display a dynamic title and dynamic content for our post like so:
Please note the:
This formats the HTML markup returned nicely and we should see something like the following:
Of course the content itself will depend on what you put in the post you created on the WordPress side and the ID in the URL bar will depend on the ID of that post.
Next we’ll want to include the SEO data as follows:
Wordpress View The Default Template
Full code for the file below:
Wordpress Vue Plugin
This has been done the simplest possible way — just to illustrate the point of how to tie it all together. I mention below how we’ve actually done quite a bit more to get this working in production.
Wrapping up
There you go, now you have a Vue.js frontend with SEO provided by Yoast’s SEO plugin from a WordPress backend!
Here’s a screenshot of our example:
Please note:
In the project referenced in the previous post we went quite a lot further and added Metadata for all taxonomies and schema.org metadata for images and videos etc.
We also used Mixins to be DRY and avoid repeating code on every different content type.
The above is actually quite important if it’s a production site but it’s tough to get all of that into one post concisely so I thought I’d explain the main mechanisms here and can cover doing this with Mixins and schema.org etc in a follow up post if there’s sufficient interest.
Wordpress View Count
What next?
Feel free to reach out if you have any trouble getting this to run — I might have missed a step.
Let me know in the comments below if you’d be interested in a follow up post or more details on this setup.
Follow me or leave a comment if you’d be keen on hearing more!
Nona designs and builds intuitive software for FinTech businesses. If you’d like to accelerate your FinTech project, book a consultation with us!
